Implementing Dynamic Unfurls in Next.js
This tutorial guides you through setting up dynamic unfurls in Next.js, showing you how to integrate Rich Unfurl dynamic images for enhanced link previews.
Posted by

Related reading
Prompt users to share content with calls to action
This tutorial is a guide on when prompt users to share content. Learn how to capitalize on the right moments to give your users the best experience.
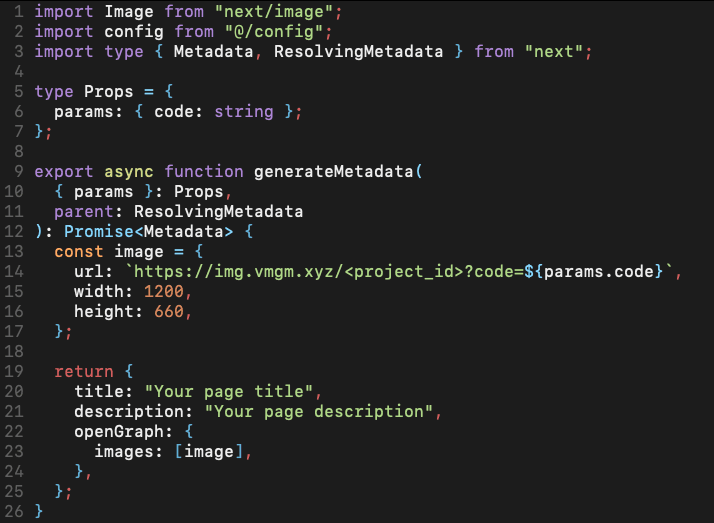
Introduction
Welcome to our guide on enhancing your Next.js application with dynamic unfurls! Today, you’ll learn how to utilize the generateMetadata function from Next.js to dynamically reference your Rich Unfurl image. Let's explore how to seamlessly integrate this feature into your project.
1. Add generateMetadata function to your page.tsx
First, copy the following code block into your page.tsx file. Then, Replace project_id with your actual project ID, which you can find on your project page.
1import type { Metadata } from "next";
2
3type Props = {
4 params: { code: string };
5};
6
7export async function generateMetadata(
8 props: Props
9): Promise<Metadata> {
10 const image = {
11 url: `https://img.vmgm.xyz/project_id?code=${props.params.code}`,
12 width: 1200,
13 height: 660,
14 };
15
16 return {
17 title: "Your page title",
18 description: "Your page description",
19 openGraph: {
20 images: [image],
21 },
22 };
23}
In this example this page is located at /app/[code]/page.tsx. This allows us to access the code variable at props.params.code. This variable can be named anything you want. You're all set to leverage Rich Unfurl in your Next.js project! Continue reading to explore additional configuration options.
2. Handle query parameters (optional)
If you want to pass more variables to your image url you can use query parameters. First, update your props type to the following.
1type Props = {
2 params: { code: string };
3 searchParams: { [key: string]: string | string[] | undefined };
4};
Now, if you have a url like https://your-domain.com?code=123&name=Chad You can access these variables likeprops.searchParams?.codeandprops.searchParams?.name and then you can use them to build your image url.3. Use your own domain for your image url (optional)
In this tutorial the image url is https://img.vmgm.xyz but you can update this to be your site domain by updating your Next config to use a rewrite. In your next.config file add the following rewrite configuration.
1/** @type {import('next').NextConfig} */
2const nextConfig = {
3 async rewrites() {
4 return [
5 {
6 source: "/img/:path*",
7 destination: "https://img.vmgm.xyz/project_id/:path*",
8 },
9 ];
10 },
11};
12
13export default nextConfig;
Now in the generateMetadata function you can replace https://img.vmgm.xyz/project_id with https://your-domain.com/imgThat wraps up our guide on using dynamic unfurls in Next.js! By integrating dynamic images, you're set to boost your project's engagement on social platforms.